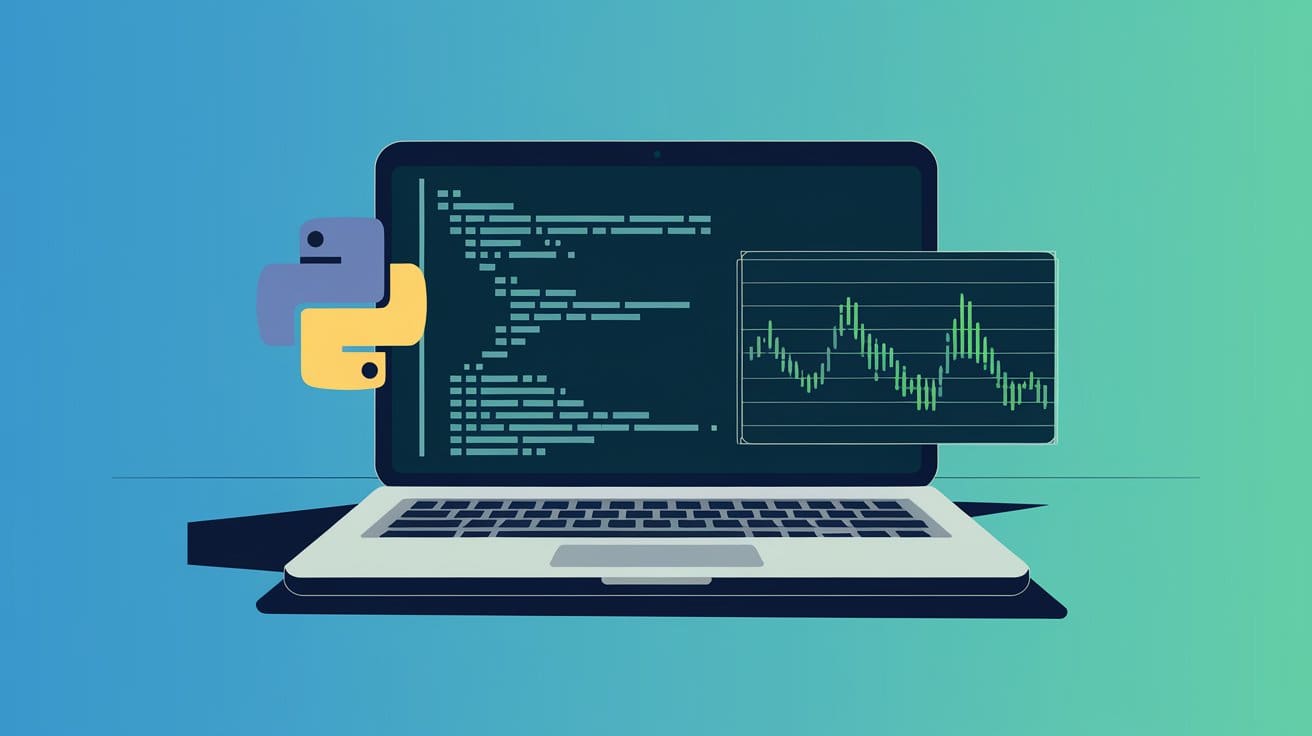
Picture by Editor | Ideogram
Let’s learn to calculate transferring averages with NumPy.
Preparation
Guarantee you have got the NumPy library put in in your surroundings. If not, you’ll be able to set up them through pip utilizing the next code:
With the NumPy library put in, we’ll be taught extra about how you can compute transferring averages within the subsequent half.
Compute Transferring Averages with NumPy
Transferring Averages (MA) is a statistical approach that creates a sequence of information factors averaged from completely different home windows of the dataset. It’s usually utilized in time-series evaluation to easy the dataset for a neater outlook on longer-term tendencies which might be onerous to see due to the short-term noises.
Transferring Averages (MAs) are sometimes used within the economic system and monetary trade to know present tendencies, forecasts, and sign indicators. The MA approach can also be thought-about a lagging indicator as a result of it’s primarily based on historic information and gives details about the present scenario.
Let’s use NumPy to compute Transferring Averages. First, we might strive calculate the Easy Transferring Common (SMA). It’s deemed so simple as it solely calculates the dataset inside the rolling home windows and takes the typical as a knowledge level.
For instance, we’ve got ten information factors for which we need to take the SMA with a window dimension of 5. We are able to do this with the next code.
import numpy as np
information = np.array([10, 15, 10, 30, 20, 45, 70, 50, 40, 60])
window_size = 5
weights = np.ones(window_size) / window_size
sma = np.convolve(information, weights, mode="legitimate")
Output>>
[17. 24. 35. 43. 45. 53.]
As we are able to see from the output, we get the transferring common with a window dimension of 5 from the information.
One other Transferring Common approach we are able to carry out is the Cumulative Transferring Common (CMA). The CMA approach would offer information factors by taking the typical of the earlier set parts of information, together with itself, for every place,
information = np.array([10, 15, 10, 30, 20, 45, 70, 50, 40, 60])
cma = np.cumsum(information) / np.arange(1, len(information) + 1)
cma
Output>>
array([10, 12.5, 11.66666667, 16.25, 17.,
21.66666667, 28.57142857, 31.2, 32.22222222, 35.])
Then, there’s an MA approach that features weight in its calculation, known as Exponential Transferring Averages (EMA). EMA provides extra weight to newer information factors than the later ones. EMA is far more delicate than SMA because it permits info on latest adjustments within the calculation. This info is represented as alpha.
Let’s strive the NumPy implementation in Python.
information = np.array([10, 15, 10, 30, 20, 45, 70, 50, 40, 60])
def exponential_moving_average(information, alpha):
ema = np.zeros_like(information)
ema[0] = information[0]
for i in vary(1, len(information)):
ema[i] = alpha * information[i] + (1 - alpha) * ema[i-1]
return ema
ema = exponential_moving_average(information, 0.5)
Output>>
array([10, 12, 11, 20, 20, 32, 51, 50, 45, 52])
That’s all for the essential NumPy implementation for computing Transferring Averages with NumPy. Attempt to grasp them to make your time-series evaluation simpler.
Further Assets
Cornellius Yudha Wijaya is a knowledge science assistant supervisor and information author. Whereas working full-time at Allianz Indonesia, he likes to share Python and information ideas through social media and writing media. Cornellius writes on quite a lot of AI and machine studying matters.
Our High 3 Associate Suggestions
1. Finest VPN for Engineers – 3 Months Free – Keep safe on-line with a free trial
2. Finest Venture Administration Device for Tech Groups – Enhance group effectivity as we speak
4. Finest Password Administration Device for Tech Groups – zero-trust and zero-knowledge safety